Basic CakePHP templating skills
Posted on 11/10/06 by Felix Geisendörfer
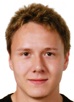
One of the things I don't see getting to much coverage is how to create good templates when working with CakePHP. Since those are written in plain PHP, this does not apply to CakePHP only. So I'm sure many people have already developed their own style that they are comfortable with and I don't ask for them to change it. However, maybe some people new to the framework / language can benifit by taking a look at the one I'm using.
PHP tags
The first thing I recommend is using the fully qualified syntax (<?php ?>) for php tags instead of the short one (<? ?>). Why? Well that's what the CakePHP coding standards recommend you to use, and it makes those statments stand out a little more in your html code. But the main reason still is that the short tags syntax can be turned off via php.ini, so using them makes your app less portable and could cause raw code output incidents.
Conditions
While you are used to use to wrap conditional commands in curly braces in your normal PHP code, they are not quite as practical in templates. Instead you should make use of this syntax which makes your code easier to read & write:
<?php else: ?>
<p>The name of the User you are looking for is: <?php echo $user['User']['name']; ?></p>
The advantage of this is that you don't have to use curly braces to wrap your command blocks, but instead simply use if, else and endif to do so.
Loops
When working with CakePHP the foreach loop is your best friend. A lot of things are done via associative arrays and even plain numerical arrays are quite comfortable to process this way. The syntax I recommend is the same one as for if statements:
<?php echo $event['Event']['html'] ?>
The linebreak issue
Now when using loops (or several inline statements) and manually viewing your html output, you'll often notice that line breaks are apparantly swallowed, and everything is on one line. Well this is not CakePHP's fault, but rather the default PHP behavior. Whenever an inline statement ends with ?> followed by a line break, this line break will not appear in the output. But don't you worry, there is a simple fix for this: Simply add a space in between ?> and the line break and voila, the output turns out as expected.
Avoid multi-line statements
One thing I found usefull when writing templates was to adobt an "avoid multi-line commands" policy. This means that you should do one command per inline statement, and not several ones at once. On occassions I break this rule, but most of the time it serves as a very good indicator for showing you that you using Controller logic in your views, or you should start writing a Helper for something. The worst thing you can do, is define functions inside your View. Not only should those be moved to helpers, but when this view get's rendered twice in one request you'll get a nasty php error, telling you you can't define the function twice ...
Creating Zebra striped table rows
This is a little specific, but I've seen some really horrible approaches for assigning varying css classes to every row in the past, so this is how I would recommend you to do it:
<td><?php echo $event['Event']['title']; ?></td>
... More columns go here ...
</tr>
Since only every 2nd number in a consecetuive series of integer values can be divided by 2 without leaving a remainder (even numbers), this can be used to constantly switch the css class between 'row-a' and 'row-b'.
Alright, I hope this little tutorial will help some new folks, and maybe some others as well.
--Felix Geisendörfer aka the_undefined